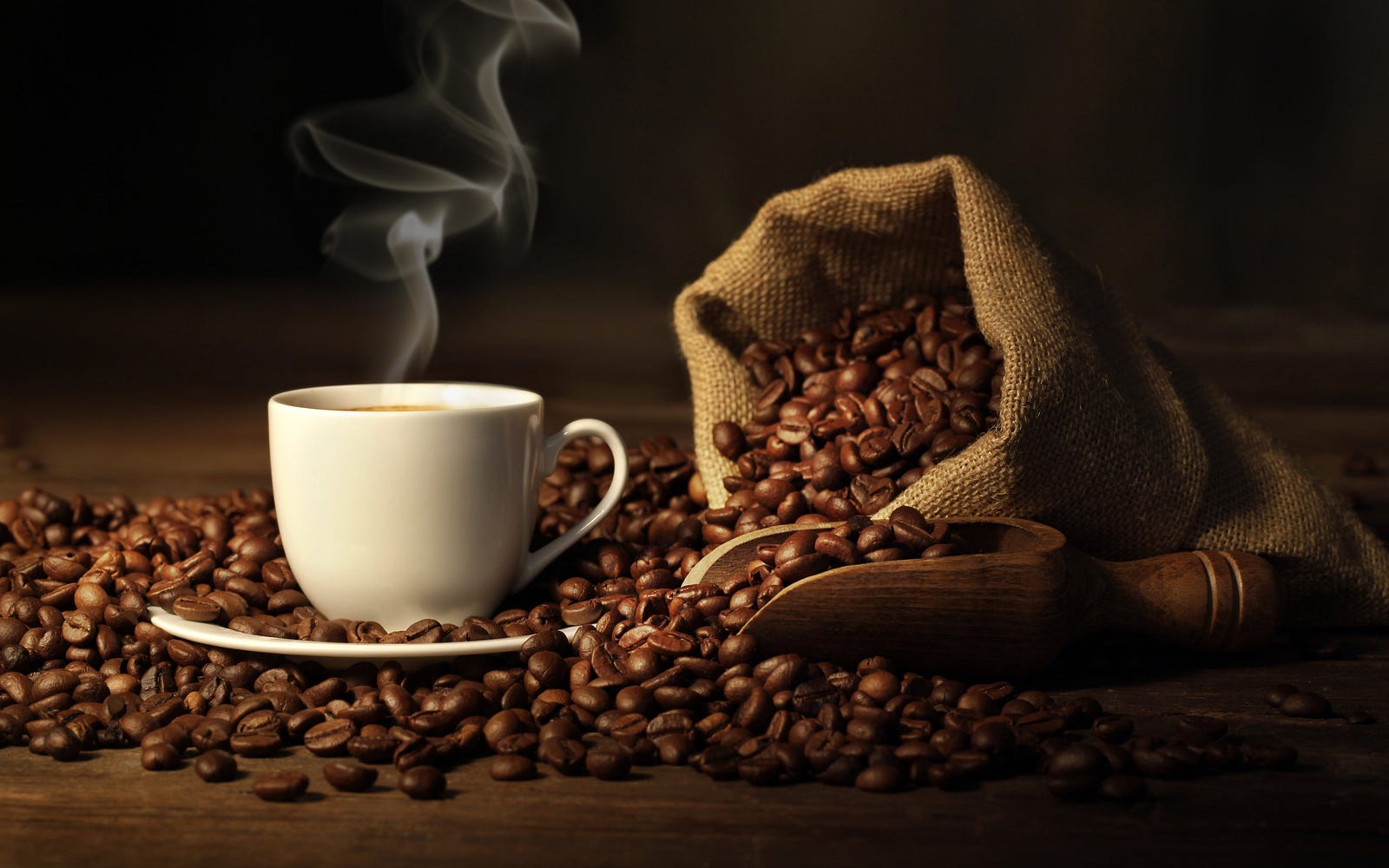
Java is a popular object-oriented programming language that offers several features to make it easier for developers to write efficient and effective code. One such feature is the “Optional” class, which was introduced in Java 8 to handle null values. Here , we will explore the Optional class in detail, explaining its purpose and demonstrating its usage with examples.
The Problem with Null Values
In Java, null is used to represent the absence of a value. However, when a variable is null and an attempt is made to access its properties or methods, a NullPointerException (NPE) occurs, which can cause the program to crash or behave unpredictably. This can be a source of bugs and errors in code.
Here is an example to explain Null Pointer Exception (NPE):
String str = null;
System.out.println(str.length());
When you run this code in main method, you’ll get a NullPointerException
because str
is null
and you're trying to access its length()
method:
Exception in thread "main" java.lang.NullPointerException at Example.main(Example.java:5)
So we need a solution. Here the solution is
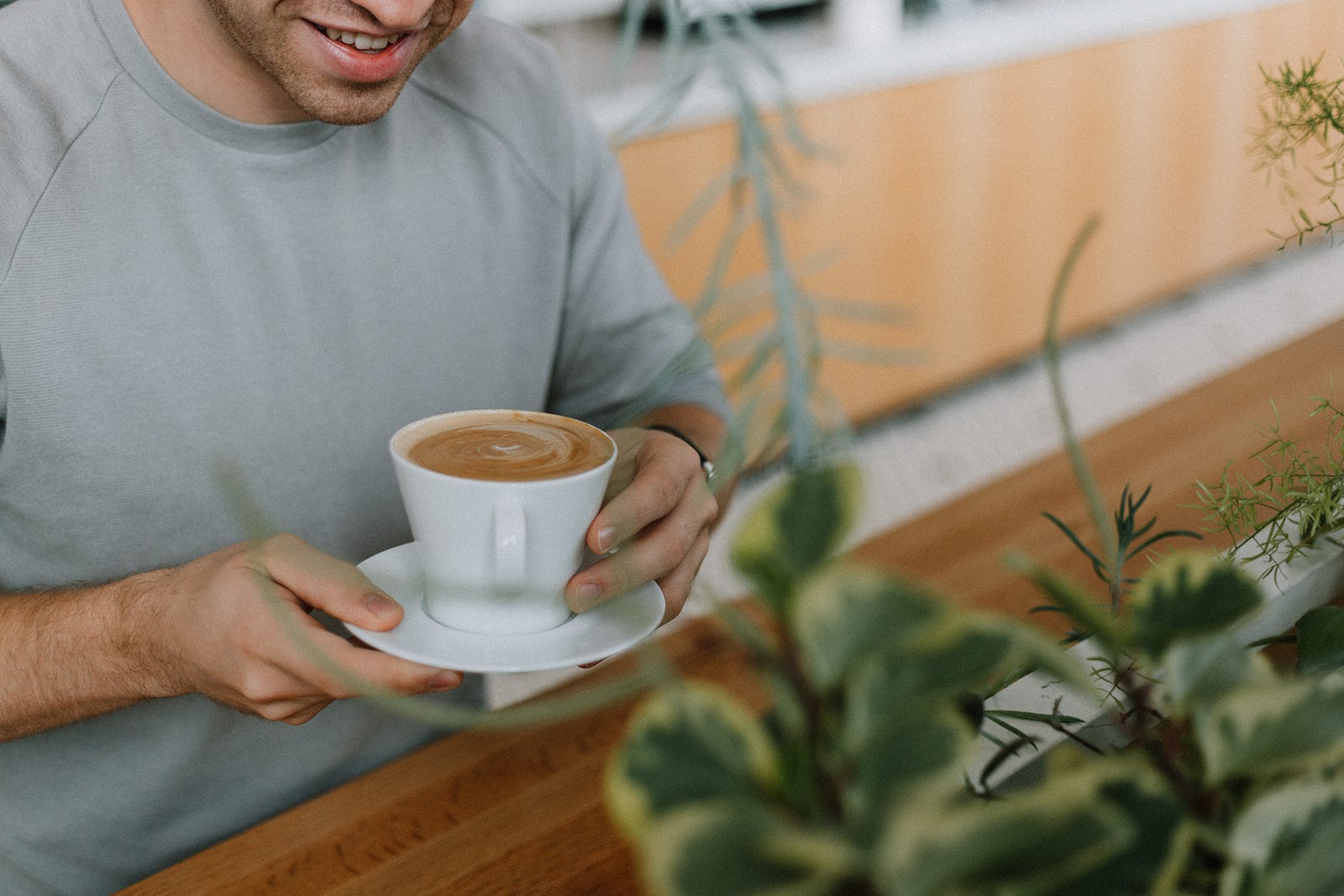
The Optional class
The Optional class was introduced to provide a solution to this problem by providing a container for values that may be null. With Optional, developers can explicitly handle null values and prevent NPEs from occurring.
It is a container that can hold either a non-null value or a null. It provides methods to check if a value is present, to retrieve the value, or to retrieve a default value if the value is null. Here is an example of how to create an Optional object:
To use Optional, you first need to import the java.util.Optional package:
import java.util.Optional;
Creating an Optional object can be done using the of()
method, which takes the value you want to wrap:
Optional<String> str = Optional.of("Bye NPE");
In this example, an Optional object is created that contains a non-null string value. If we wanted to create an Optional object that may contain null, we could use the Optional.empty()
method instead
Optional<String> str = Optional.empty();
Alternatively, we could use the Optional.ofNullable()
method, which creates an Optional object that may or may not contain null:
String str = null;
Optional<String> str = Optional.ofNullable(str);
Lets’s dive deeper,
Once an Optional object is created, we can use its methods to retrieve the value or handle the case where the value is null. Here are some examples:
Optional<String> str = Optional.of("Hello, world!");
System.out.println(str.isPresent());// true
str.ifPresent(str1-> System.out.println(str1.length())); //13
System.out.println(str.get()); // "Hello, world!"
Optional<String> emptystr = Optional.empty();
System.out.println(emptystr.isPresent());// false
emptystr.ifPresent(str2-> System.out.println(str2.length()));//skip this line
System.out.println(emptystr.orElse("default"));// "default"
String nullStr = null;
Optional<String> nullablestr = Optional.ofNullable(nullStr);
System.out.println(nullablestr.isPresent()); //false
System.out.println(nullablestr.orElse("default")); // "default"
the isPresent()
method to check if a value is present,and ifPresent()
method to check if a value is presentthen do something and the get()
method to retrieve the value.
In the second example, we create an empty Optional object and use the isPresent()
method to check if a value is present. We use ifPresent()
also help to do something if it is not null, that's why the the line of code was skipped. We also use the orElse()
method to retrieve a default value if the Optional object contains null.
In the third example, we create an Optional object that may or may not contain null. We use the isPresent()
method to check if a value is present, and the orElse()
method to retrieve a default value if the Optional object contains null.
Okay, Enough
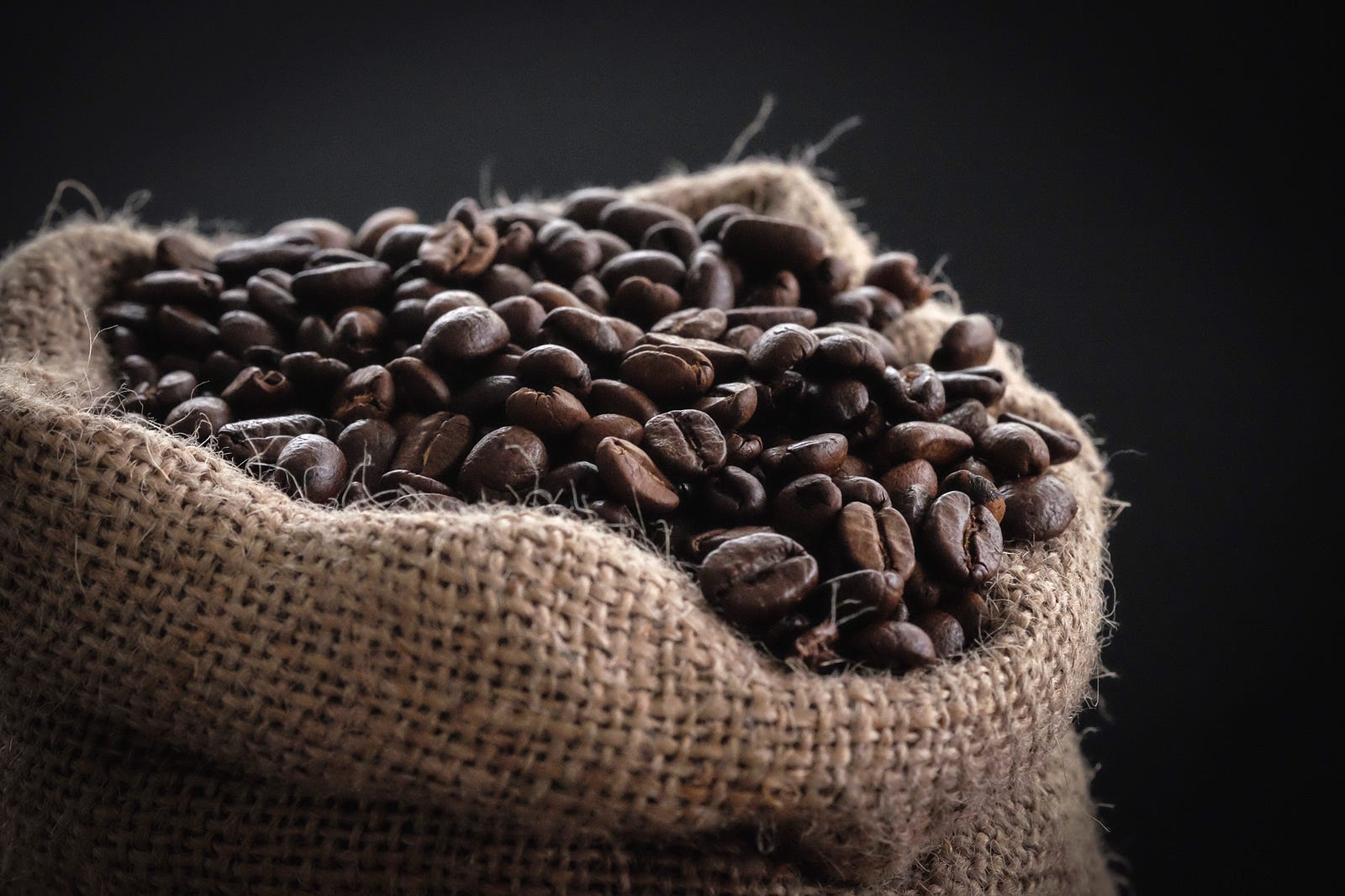
Come to the conclusion
Overall, Optional is a powerful tool for handling null values in Java and making code more robust. It provides methods to check if a value is present, to retrieve the value, or to retrieve a default value if the value is null. By using Optional, developers can write cleaner and more efficient code, reducing the likelihood of bugs and errors.
OKay Bye !!!
Hope you will enjoy.
Leave a comment below or ask me via twitter and follow me on insta if you have any questions https://twitter.com/Srkaran7. https://www.instagram.com/karan_s_r_/?hl=en
0 Comments